A reader recently asked me about some troubles he was having getting my 32-bit floating point package up and running. I thought it would be a great test for my new VS Code 65816 debugging extension. With just a few edits to the test program the reader was using, I was up and running in the VS Code debugger.
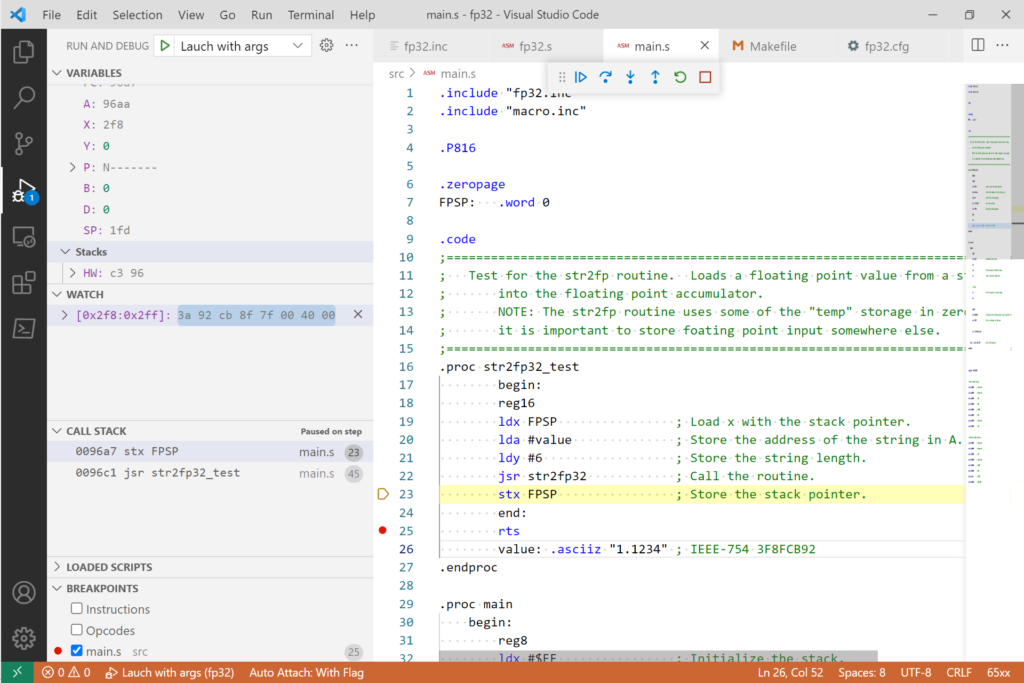
The reader’s test program simply adds the floating-point value 1.1234 to the floating-point stack. The IEEE-754 representation of that value is 3F8FCB92. A handy online calculator confirms this. You can see this at the top of the floating-point stack in the Watch pane in the image above (I won’t get into the internal representation of floating-point values in the fp32 package, but take my word for it, they are the same).
You can try it out as well using the cc65 assembler. Here is the test code (only lightly edited from the readers file):
main.s
.include "fp32.inc"
.include "macro.inc"
.P816
.zeropage
FPSP: .word 0
.code
;==============================================================================
; Test for the str2fp routine. Loads a floating point value from a string
; into the floating point accumulator.
; NOTE: The str2fp routine uses some of the "temp" storage in zero page. So
; it is important to store foating point input somewhere else.
;==============================================================================
.proc str2fp32_test
begin:
reg16
ldx FPSP ; Load x with the stack pointer.
lda #value ; Store the address of the string in A.
ldy #6 ; Store the string length.
jsr str2fp32 ; Call the routine.
stx FPSP ; Store the stack pointer.
end:
rts
value: .asciiz "1.1234" ; IEEE-754 3F8FCB92
.endproc
.proc main
begin:
reg8
ldx #$FF ; Initialize the stack.
txs
cld ; Clear decimal arithmetic mode.
cli ; Clear interrupt enable bit.
; native
clc ; shift processor to native mode
xce
reg16
ldx #$0300 ; Initialize the floating point stack register.
stx FPSP ; This is defined in fp32.asm
jsr str2fp32_test
exit: .byte $42,$FF ; Exits the emulator.
.endproc
.segment "VECTORS"
; Native Mode Vectors
.word $0000 ; Reserved
.word $0000 ; Reserved
.word $0000 ; COP
.word $0000 ; BRK
.word $0000 ; ABORT
.word $0000 ; NMI
.word $0000 ; Reserved
.word $0000 ; IRQ
; Emulation Mode Vectors
.word $0000 ; Reserved
.word $0000 ; Reserved
.word $0000 ; COP
.word $0000 ; Reserved
.word $0000 ; ABORT
.word $0000 ; NMI
.word main ; RESET
.word $0000 ; IRQ/BRK
Here is the configuration file:
fd32.cfg
MEMORY
{
ZP: start=$0000, size=$0100, type=rw, fill=yes, fillval=$0, define=yes;
STACK: start=$0100, size=$0100, type=rw, fill=yes, fillval=$0, define=yes;
RAM: start=$0200, size=$7E00, type=rw, fill=yes, fillval=$0, define=yes;
ROM: start=$8000, size=$8000, type=ro;
}
SEGMENTS
{
ZEROPAGE: load=ZP, type=zp;
BSS: load=RAM, type=rw;
RODATA: load=ROM, type=ro, start=$8000;
CODE: load=ROM, type=ro, start=$9000;
VECTORS: load=ROM, type=ro, start=$ffe0;
}
Here is my NMake file:
Makefile
# check https://docs.microsoft.com/en-us/cpp/build/reference/nmake-reference?view=msvc-160
# to simplify
E = c:\cc65
TARGET = fp32.bin
W = C:\Users\tmrob\Documents\Projects\GitHub\testing\local\fp32
R = $W\Release
O = $R\obj
S = $W\src
L = $R\listing
SOURCES = $S\fp32.s $S\main.s
OBJECTS = $O\fp32.o $O\main.o
all : $(TARGET)
$(TARGET) : $(OBJECTS) $(SOURCES) $R\Makefile $R\fp32.cfg $S\macro.inc
$E\ca65 --cpu 65816 -g -l $L\fp32.lst -o $O\fp32.o $S\fp32.s
$E\ca65 --cpu 65816 -g -l $L\main.lst -o $O\main.o $S\main.s
$E\ld65 -o $(TARGET) -m $L\fp32.map -Ln $L\fp32.sym -C $R\fp32.cfg --dbgfile $L\fp32.dbg $(OBJECTS)
And finally, here is the launch file I used to start debugging in the VS Code db65xx extension:
launch.json
{
"version": "0.2.0",
"configurations": [
{
"type": "65xx",
"request": "launch",
"name": "Lauch with args",
"program": "${cwd}",
"args": [
{
"sbin": "${cwd}\\Release\\fp32.bin",
"src": "${cwd}\\src",
"list": "${cwd}\\Release\\listing",
"input": "0xf004",
"output": "0xf001"
}
],
"stopOnEntry": true,
"cwd": "${cwd}"
}
]
}
Of course, you’ll need to edit these for the location of your own files but with that you should be up and running. Try it out and have fun modifying it. If run as is, make sure to set a breakpoint at the end of the str2fp32_test routine as I haven’t modified the reader’s code to pause or gracefully end.
If you run into any problems, drop me a line at trobertson@trobertson.site or ask a question at my GitHub. If you have a general question about using the db65xx extension, you can ask it here.